UseFactory Attribute added to Laravel
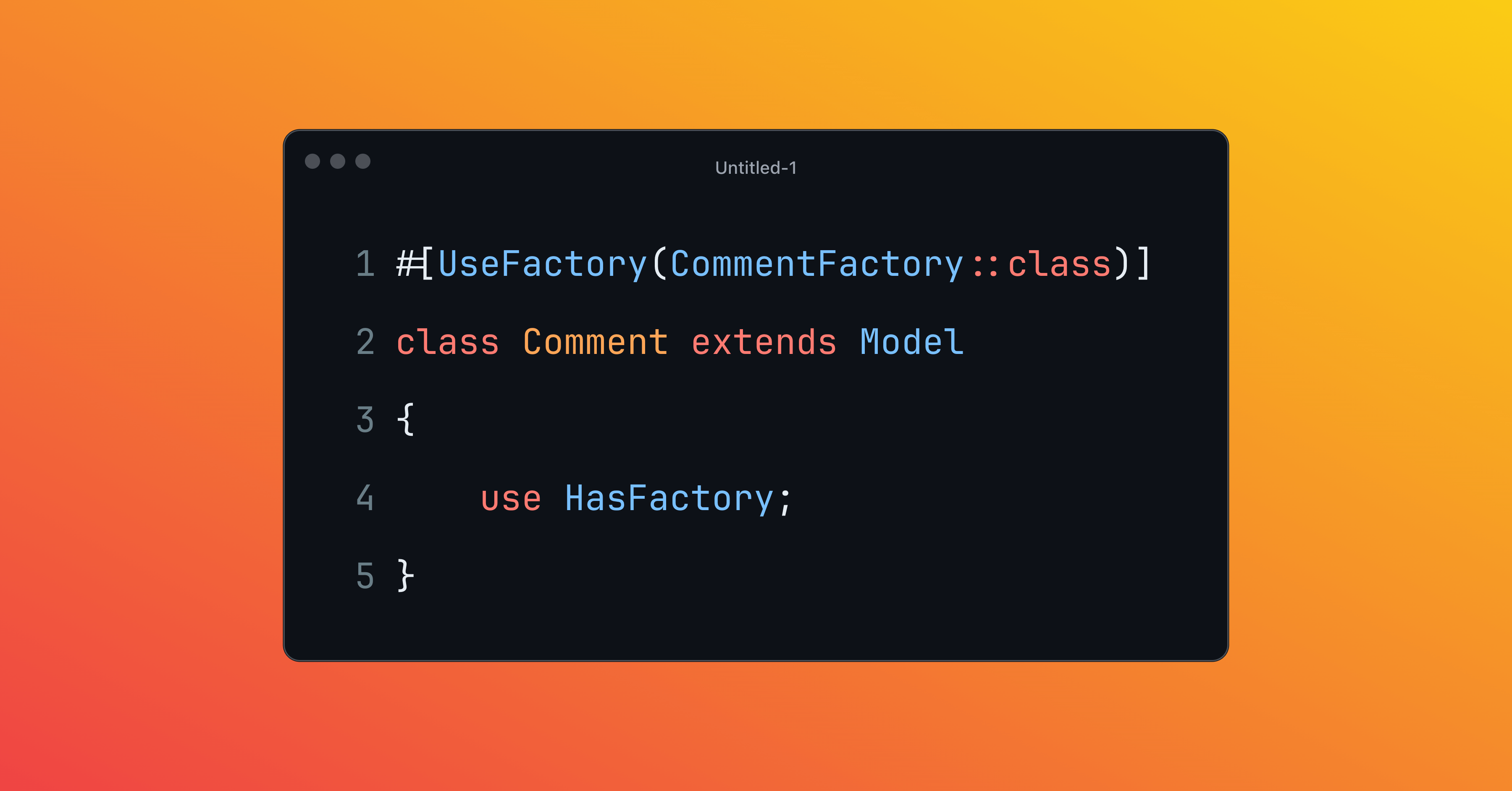
27th January 2025
Associating a Factory with a Model
Last week saw another minor release of the framework, one feature that stood out to me was small but quite valuable.
Here is an example from the [pull request by Christopher Arter
<?php
namespace App\Domain\Models\Comment;
use Database\Factories\CommentFactory;
use Illuminate\Database\Eloquent\Relations\HasMany;
use Illuminate\Database\Eloquent\Attributes\UseFactory;
#[UseFactory(CommentFactory::class)]
class Comment extends Model
{
use HasFactory;
// ...
}
Usually, when we use the HasFactory
trait on a model, it will use conventions to determine the associated factory.
It will search the Database\Factories
namespace that has a class name matching the model name and suffixed with Factory
In some cases, a project may not be structured in a conventional way, meaning that we would have to add a newFactory()
method to a model and return an instance of the models corresponding factory.
<?php
namespace App\Domain\Models\Comment;
use Database\Factories\CommentFactory;
use Illuminate\Database\Eloquent\Relations\HasMany;
use Illuminate\Database\Eloquent\Attributes\UseFactory;
class Comment extends Model
{
use HasFactory;
protected static function newFactory()
{
return CommentFactory::new();
}
// ...
}
The new attribute is really handy as a replacement for this method on the model, we can now just add the attribute to the top of the class.
<?php
#[UseFactory(CommentFactory::class)]
A small but pretty nice change, I imagine we will be seeing quite a few of these types of improvements over the next few months.